Html Web Pages Generator with Python And ChatGPT
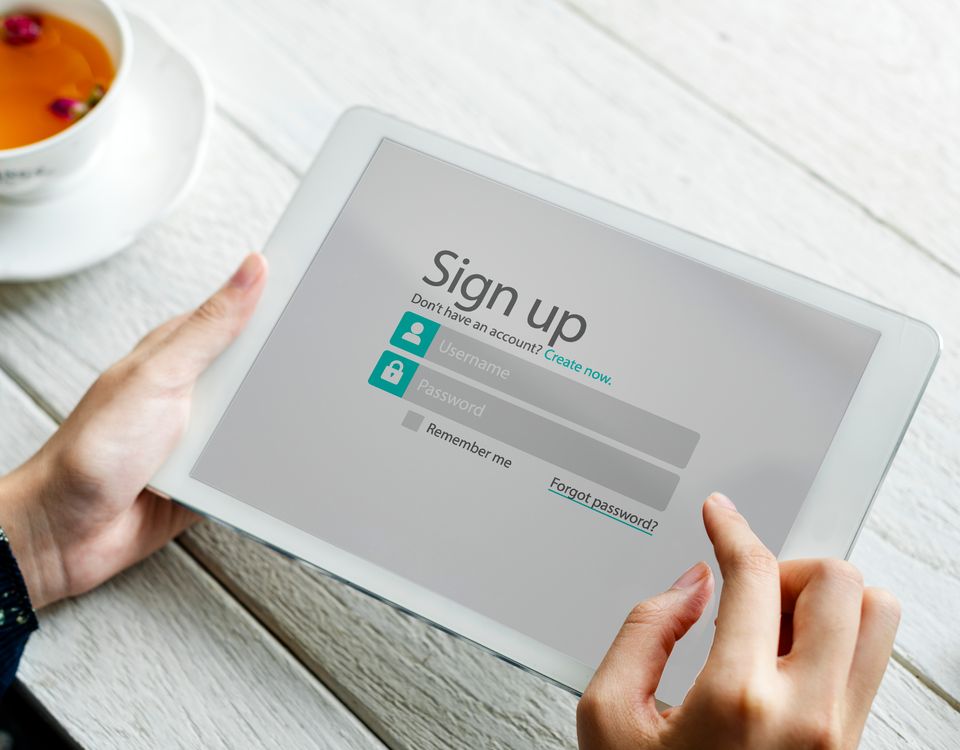
Creating web pages from scratch can be a tedious job. Fortunately, our new friend "Generative Pre-trained Transformer 3" also known as ChatGPT can help with that as well. In this simple python tutorial, you'll learn how to generate HTML pages and open them automatically in your local browser.
Prerequisites:
- Install python 3 on your machine.
- Signup to OpenAI API and get your API key (under "Profile" -> "View API keys")
Setup Python Env
Go ahead and create a new python project on your preferred IDE, I'm using Pycharm in this example but you can use whichever IDE you would like.
The first thing we need to do is to import OpenAI library for us to use in our python code.
To install OpenAI library use the following pip command pip3 install openai
.
Once installed import it to your main.py file.
Before we can use the API we need to set our API key for our requests, it is done by setting openai.api_key
, in the snippet below I loaded it from an environment variable to not expose my own API key, but you can paste your key as is.
import openai
openai.api_key = os.getenv('OPEN_AI_API_KEY')
def generate_html():
print("generate an amazing site for me!")
if __name__ == '__main__':
generate_html()
Understanding OpenAI API Properties
We can now start exploring the API functionalities.
def generate_plain_response(prompt):
print(prompt)
completion = openai.Completion.create(
engine=MODEL_ENGINE,
prompt=prompt,
max_tokens=MAX_TOKENS,
temperature=0.5,
frequency_penalty=0,
presence_penalty=0
)
return completion.choices[0].text
this is the generic call to the API for text completion. Let's walk over its properties.
- engine: The model id you would like to use in your request. In this example, I used "text-davinci-003" latest model when creating this blog post.
- prompt: The prompt to generate completion for (obviously)
- max_tokens: The maximum number of tokens to generate in the completion.
- temperature: Higher values like 0.8 will make the output more random, while lower values like 0.2 will make it more focused and deterministic.
- frequency_penalty: positive values will decrease the model's likelihood to repeat the same line verbatim.
- presence_penalty: positive values will increase the model's likelihood to talk about new topics
Creating Our Prompt
So we want to generate a website, and what is more exciting than a website on cats?? Let's do just that!
In our prompts to ChatGPT/OpenAI API, we have to be very specific and precise in describing exactly how we want the output to look like.
Look at this prompt for example:
prompt = "create an html web page for a site about breeds of cats with bootstrap 5. the site should have " \
"a bootstarp nav bar including CatBreeds brand name, about, contact and blog. the first section should represent the site" \
"idea and visionand the passion of the owner about cats. the second section should contain" \
"a bootstrap carousel with 3 breads of cats each with its photo from picsum.photos and description, the carousel should have " \
"navigation buttons. " \
"the last section should contain a footer with all the contact information." \
"style the site with css to make it approachable and convenient."
Notice how I mentioned "Bootsrap 5" for the website layout and the different sections I described (a navbar, a carousel, and a footer). If your prompts will lack these descriptions you will get fairly random unwanted results.
Sending A Request
Let's make our first call and see if everything works as expected.
html_content = generate_plain_response(prompt)
When experimenting with the API I noticed that sometimes the response cut off and the HTML page is not complete.
I managed to bypass this issue by creating a "feedback-loop" to the model.
The idea is to send the HTML content back for the model to evaluate. If the HTML page is valid, we will ask it to reply with "Yes" or else complete the missing pieces.
Feedback loop
First, we need to create the feedback loop function
def html_feedback_loop(prompt, html):
response = generate_response_with_context(prompt, html)
print(response)
return response
Then we need to call it from a function that generates the HTML
def generate_html():
html_content = generate_plain_response(prompt)
for i in range(0, 10):
answer = html_feedback_loop(html_content,
"if the html in the context is valid return Yes else complete the missing parts in the page")
if "Yes" in answer:
break
else:
html_content = answer
return html_content
Checking for the word "Yes" is our way of making sure the output is indeed complete. Since the model is not consistent in the way it answers, from my experience I found out that the word yes is always within the 5th words chunk.
Notice, there is a token limit that can be sent to the model. Sometimes, if the HTML content is too big you will get a failure. We can solve this issue by requesting different sections and patching them together on our own, but that is a topic for another blog post.
Writing The Content To A File
We will use the simple .write() python function to save the generated content to a .html file. I added UUID to each file in order to not override previous results.
import uuid
def save_html_file(content):
html_file_name = 'mySite' + str(uuid.uuid4()) + '.html'
with open(html_file_name, 'w') as f:
f.write(content)
return html_file_name
Automatically Open The HTML file Once Saved
In order to open a file in a web browser from our python application we need to use webbrowser library
import webbrowser
def open_file_in_browser(file_name):
filename = 'file:///' + os.getcwd() + '/' + file_name
webbrowser.open_new_tab(filename)
Results
Here are some samples of the results I got when writing this post.
CatBreeds
CatBreeds is a website dedicated to the appreciation of cats and their many breeds. We strive to provide the latest information on all the different breeds of cats and their characteristics. We also provide advice on how to care for cats and how to choose the right breed for you. We are passionate about cats and the joy they bring to our lives. We hope you will find our website to be a great source of information on all the different breeds of cats and the wonderful companions they can be.
CatBreeds
Welcome to CatBreeds! We are passionate about cats and their many breeds. We have a great selection of cats from all over the world. We are dedicated to helping you find the perfect breed of cat for your home. Whether you are looking for a lap cat, a playful cat, or a cuddly companion, we have the perfect breed for you. We are committed to providing you with the best possible care and guidance when it comes to choosing the right breed for you and your family. We have a wealth of knowledge and experience when it comes to cats and their many breeds. We are here to help you make an informed decision when it comes to choosing the right breed of cat for your home. Thank you for visiting CatBreeds!
Conclusion
You can see that the results are far from perfect, but that is a huge kickstart for any front-end project you can come up with. You can take the output and start editing it for your needs right away.
The whole code for this project can be found on my GitHub page.